Java Core
May 21, 2023 2023-06-25 19:09Java Core
Java Core
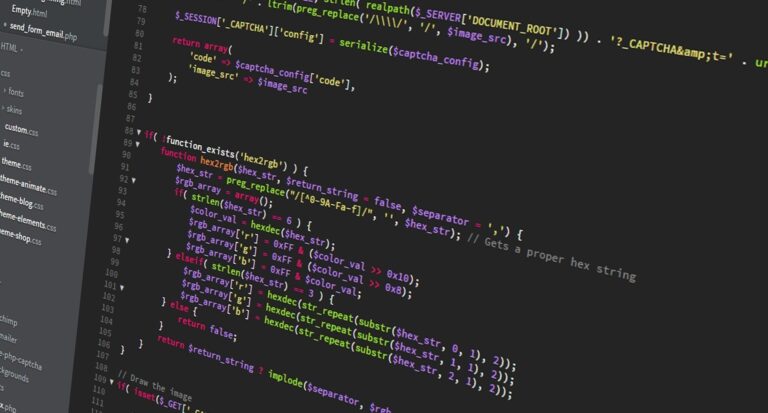
Our Modules
1.1 Overview of Java
1.2 Java Development Environment Setup
1.3 Basic Structure of a Java Program
1.4 Variables and Data Types
1.5 Operators in Java
1.6 Control Flow Statements (if-else, switch-case, loops)
2.1 Introduction to Object-Oriented Programming (OOP)
2.2 Classes and Objects
2.3 Inheritance and Polymorphism
2.4 Encapsulation and Access Modifiers
2.5 Abstract Classes and Interfaces
2.6 Packages and Import Statements
3.1 Introduction to Collections Framework
3.2 ArrayList, LinkedList, and Vector
3.3 HashSet, LinkedHashSet, and TreeSet
3.4 HashMap, LinkedHashMap, and TreeMap
3.5 Queue and Deque
3.6 Iterator and ListIterator
4.1 Exception Handling Basics
4.2 Try-Catch Blocks and Exception Types
4.3 Throwing and Catching Exceptions
4.4 Handling Multiple Exceptions
4.5 File Input and Output (I/O) in Java
4.6 Reading and Writing Files
5.1 Introduction to Multithreading
5.2 Creating and Running Threads
5.3 Thread Synchronization and Interthread Communication
5.4 Thread Pools and Executors
5.5 Java Concurrent Collections
5.6 Atomic Variables and Locks
6.1 Introduction to Java GUI Programming
6.2 Swing Components (JFrame, JLabel, JButton, etc.)
6.3 Event Handling and Listener Interfaces
6.4 Layout Managers (FlowLayout, BorderLayout, GridBagLayout)
6.5 Menus and Dialog Boxes
6.6 Introduction to JavaFX (optional)
7.1 Generics in Java
7.2 Lambda Expressions and Functional Interfaces
7.3 Streams and Stream API
7.4 Java Database Connectivity (JDBC)
7.5 Java Serialization
7.6 Java Reflection